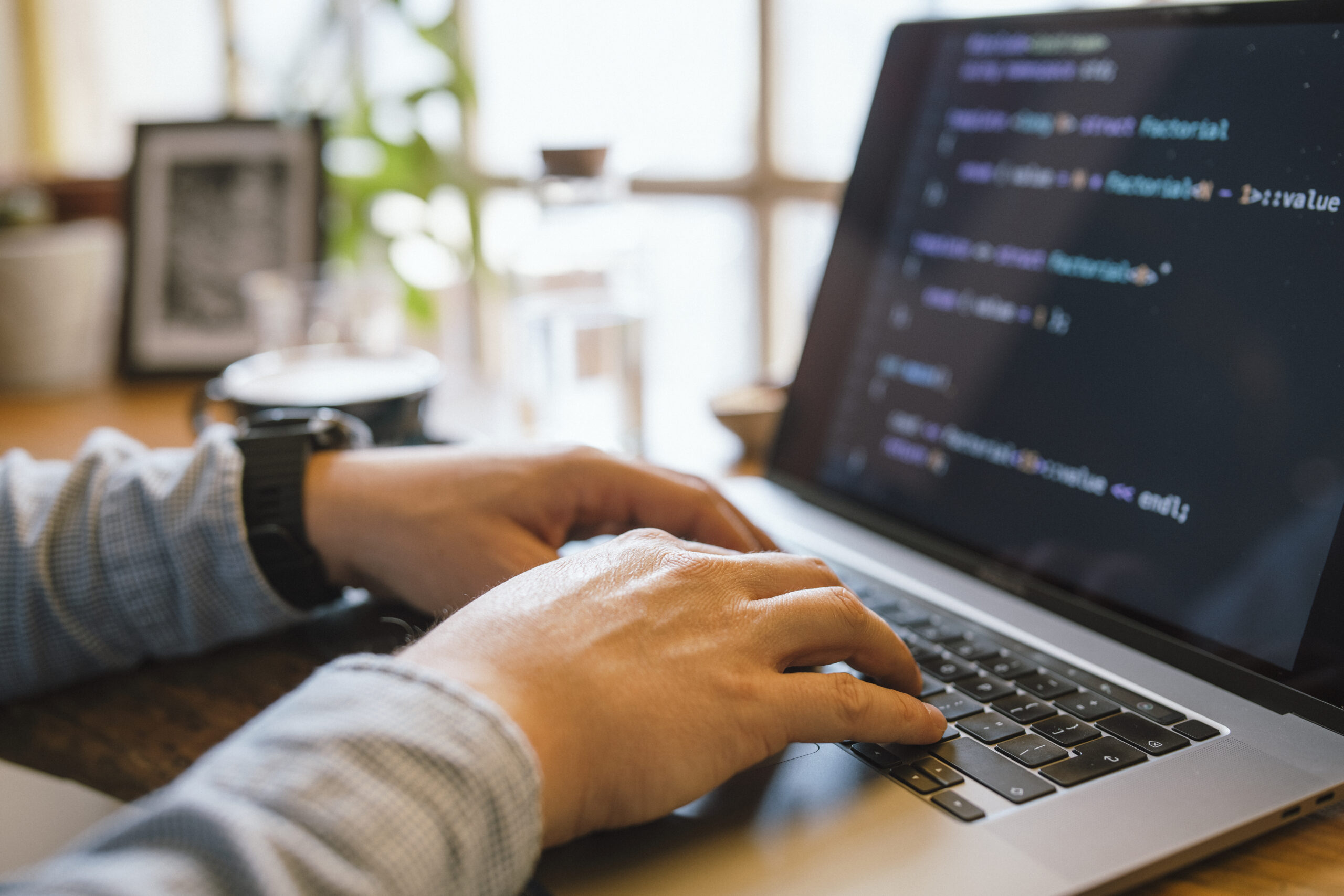
Debugging is Just about the most essential — but generally missed — skills inside a developer’s toolkit. It is not nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and Discovering to think methodically to solve problems efficiently. Regardless of whether you're a newbie or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of aggravation and significantly enhance your productivity. Listed here are several strategies to help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
One of the fastest strategies developers can elevate their debugging abilities is by mastering the tools they use everyday. Though producing code is a single A part of development, recognizing tips on how to communicate with it successfully during execution is Similarly crucial. Modern enhancement environments appear equipped with highly effective debugging capabilities — but many builders only scratch the surface of what these applications can do.
Just take, as an example, an Built-in Growth Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, stage through code line by line, and in some cases modify code around the fly. When utilized properly, they Enable you to observe accurately how your code behaves in the course of execution, which is priceless for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-finish builders. They enable you to inspect the DOM, keep track of community requests, see serious-time functionality metrics, and debug JavaScript within the browser. Mastering the console, resources, and network tabs can switch frustrating UI concerns into workable tasks.
For backend or technique-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage about operating processes and memory administration. Learning these resources could possibly have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn out to be relaxed with Model Command systems like Git to comprehend code heritage, obtain the precise moment bugs were introduced, and isolate problematic adjustments.
Eventually, mastering your instruments suggests heading over and above default options and shortcuts — it’s about producing an personal expertise in your enhancement ecosystem so that when problems arise, you’re not lost at midnight. The better you realize your resources, the more time you are able to invest solving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the situation
Among the most important — and sometimes disregarded — measures in efficient debugging is reproducing the problem. Before leaping to the code or producing guesses, developers need to produce a regular surroundings or scenario where the bug reliably seems. With no reproducibility, fixing a bug becomes a video game of possibility, frequently bringing about squandered time and fragile code modifications.
The initial step in reproducing a challenge is collecting just as much context as is possible. Request questions like: What steps led to The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it gets to be to isolate the precise circumstances less than which the bug happens.
As you’ve collected more than enough information and facts, try and recreate the problem in your neighborhood environment. This might necessarily mean inputting precisely the same data, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, take into account writing automated assessments that replicate the sting instances or point out transitions involved. These exams not simply help expose the challenge but will also avoid regressions Sooner or later.
Sometimes, the issue could possibly be environment-certain — it would materialize only on particular working devices, browsers, or less than specific configurations. Employing applications like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the problem isn’t just a stage — it’s a frame of mind. It necessitates tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you might be now midway to correcting it. Which has a reproducible scenario, You should use your debugging resources a lot more efficiently, examination likely fixes safely and securely, and converse far more Plainly using your crew or end users. It turns an abstract complaint into a concrete challenge — Which’s where by builders prosper.
Examine and Fully grasp the Mistake Messages
Error messages are frequently the most precious clues a developer has when some thing goes Incorrect. Instead of seeing them as disheartening interruptions, builders need to find out to treat mistake messages as immediate communications from your method. They often show you just what exactly took place, in which it happened, and in some cases even why it took place — if you know how to interpret them.
Start by examining the concept cautiously As well as in entire. Several builders, particularly when below time tension, glance at the very first line and straight away start off creating assumptions. But further inside the mistake stack or logs may possibly lie the accurate root induce. Don’t just copy and paste mistake messages into serps — go through and have an understanding of them 1st.
Break the mistake down into parts. Could it be a syntax mistake, a runtime exception, or simply a logic error? Will it stage to a certain file and line quantity? What module or purpose triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also handy to comprehend the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Studying to acknowledge these can greatly quicken your debugging course of action.
Some errors are vague or generic, As well as in Those people situations, it’s very important to examine the context through which the mistake happened. Verify relevant log entries, enter values, and up to date modifications while in the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede greater difficulties and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns speedier, cut down debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Just about the most strong instruments in a very developer’s debugging toolkit. When applied correctly, it offers serious-time insights into how an software behaves, encouraging you have an understanding of what’s going on underneath the hood without having to pause execution or move in the code line by line.
A very good logging system starts off with figuring out what to log and at what stage. Widespread logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts through growth, Data for basic occasions (like successful get started-ups), Alert for likely concerns that don’t break the applying, ERROR for real problems, and Lethal once the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure vital messages and decelerate your method. Focus on critical functions, state improvements, input/output values, and important final decision points inside your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re In particular beneficial in output environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. By using a perfectly-believed-out logging tactic, you can decrease the time it will require to identify concerns, get further visibility into your applications, and Enhance the Over-all maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not just a technical process—it is a method of investigation. To effectively recognize and deal with bugs, builders will have to method the method just like a detective fixing a thriller. This way of thinking helps break down complicated concerns into workable areas and abide by clues logically to uncover the foundation cause.
Begin by gathering evidence. Look at the signs and symptoms of the challenge: error messages, incorrect output, or functionality difficulties. The same as a detective surveys against the law scene, obtain just as much suitable facts as you could without the need of leaping to conclusions. Use logs, exam scenarios, and person stories to piece jointly a clear image of what’s taking place.
Subsequent, form hypotheses. Ask yourself: What could be producing this behavior? Have any changes a short while ago been built to your codebase? Has this situation transpired prior to under identical instances? The target is always to narrow down possibilities and detect likely culprits.
Then, check your theories systematically. Attempt to recreate the condition in a very controlled environment. For those who suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Just like a detective conducting interviews, inquire your code questions and Permit the outcomes guide you closer to the reality.
Shell out close awareness to little aspects. Bugs typically hide from the least envisioned areas—similar to a missing semicolon, an off-by-a person mistake, or maybe a race problem. Be complete and individual, resisting the urge to patch The difficulty with no fully knowledge it. Short-term fixes may well hide the true problem, just for it to resurface afterwards.
Finally, continue to keep notes on Everything you tried out and realized. Equally as detectives log their investigations, documenting your debugging process can preserve time for upcoming concerns and enable Other people fully grasp your reasoning.
By thinking just like a detective, builders can sharpen their analytical skills, strategy complications methodically, and turn out to be simpler at uncovering concealed challenges in sophisticated devices.
Write Exams
Composing assessments is among the most effective methods to increase your debugging techniques and overall improvement effectiveness. Assessments don't just assistance catch bugs early but additionally serve as a safety Internet that provides you self esteem when earning changes for your codebase. A effectively-examined application is much easier to debug mainly because it allows you to pinpoint precisely in which and when a difficulty happens.
Begin with unit exams, which concentrate on personal functions or modules. These little, isolated exams can rapidly reveal whether or not a specific piece of logic is Operating as expected. When a test fails, you immediately know where to glimpse, noticeably lessening enough time put in debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear immediately after Earlier getting fixed.
Future, combine integration exams and finish-to-finish checks into your workflow. These enable be certain that different parts of your software operate with each other smoothly. They’re specially beneficial for catching bugs that happen in elaborate programs with several factors or expert services interacting. If one thing breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing tests also forces you to definitely Believe critically regarding your code. To test a element effectively, you would like to grasp its inputs, expected outputs, and edge situations. This level of knowledge Normally sales opportunities to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. When the test fails constantly, you could give attention to correcting the bug and watch your examination go when The difficulty is resolved. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the disheartening guessing sport into a structured and predictable course of action—helping you catch a lot more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult challenge, it’s quick to be immersed in the issue—looking at your display for hrs, hoping Alternative after Answer. But Just about the most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your thoughts, minimize stress, and sometimes see The problem from a new viewpoint.
When you are also near to the code for also extended, cognitive tiredness sets in. You could commence overlooking clear mistakes or misreading code which you wrote just hours earlier. Within this state, your Mind results in being fewer successful at challenge-fixing. A short walk, a espresso crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your aim. Quite a few developers report discovering the root of a dilemma when they've taken time for you to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, especially all through extended debugging periods. Sitting down before a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Vitality and a clearer way of thinking. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
In the event you’re trapped, an excellent general guideline is usually to set a timer—debug actively for 45–sixty minutes, then take a five–10 moment break. Use that point to maneuver all around, extend, or do anything unrelated to code. It may come to feel counterintuitive, especially underneath tight deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
In brief, having breaks isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel vision that often blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Discover From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—it's a chance to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, each one can teach you one thing worthwhile for those who make an effort to reflect and examine what went Mistaken.
Start out by inquiring you a couple of crucial queries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved practices like device tests, code reviews, or logging? The answers often expose blind places in the workflow or understanding and help you build stronger coding habits going forward.
Documenting bugs can also be an excellent habit. Keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or prevalent problems—which you can proactively stay get more info away from.
In team environments, sharing what you've acquired from the bug along with your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast information-sharing session, assisting Many others stay away from the exact same issue boosts staff efficiency and cultivates a much better Finding out culture.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, some of the finest developers are certainly not the ones who publish perfect code, but people that constantly study from their errors.
In the long run, each bug you deal with provides a fresh layer towards your skill established. So future time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging techniques takes time, follow, and endurance — but the payoff is huge. It would make you a far more effective, assured, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become far better at That which you do.